JavaScript ES6+: 10 Features Every Front-End Developer Should Know
JavaScript has evolved significantly over the years, with ES6 (ECMAScript 2015) introducing many features that have transformed the way developers write code. If you're a front-end developer or JavaScript Developer, understanding these features is essential for writing cleaner, more efficient, and maintainable code. In this post, we’ll explore 10 must-know ES6+ features that every web developer should be familiar with.
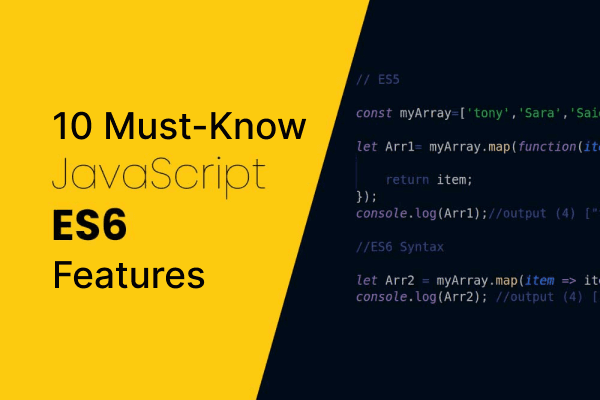
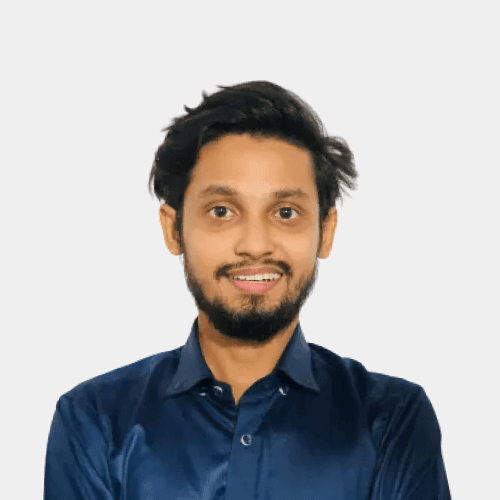
Md Kayesh
6 min readAugust 16, 2024
1. Arrow Functions
Arrow functions offer a more concise way to write functions in JavaScript. They also have a unique feature: they don’t bind their own `this` value, which can be particularly useful in certain contexts like event handlers and callbacks.
// Traditional function
function add(a, b) {
return a + b;
}
// Arrow function
const add = (a, b) => a + b;
2. Template Literals
Template literals allow you to embed variables and expressions directly into strings, making string interpolation easier and more readable.
const name = 'Alice';
const greeting = `Hello, ${name}! Welcome to ES6+ features.`;
3. Destructuring Assignment
Destructuring lets you unpack values from arrays or properties from objects into distinct variables. This simplifies the extraction of values from complex data structures.
// Array destructuring
const [first, second] = [1, 2];
// Object destructuring
const { name, age } = { name: 'Bob', age: 25 };
4. Default Parameters
With default parameters, you can specify default values for function parameters, making your code more robust and preventing `undefined` errors.
function greet(name = 'Guest') {
return `Hello, ${name}!`;
}
5. Rest and Spread Operators
The rest operator (`...`) allows you to represent an indefinite number of arguments as an array. The spread operator spreads elements of an array or object into individual elements.
// Rest operator
function sum(...numbers) {
return numbers.reduce((total, num) => total + num, 0);
}
// Spread operator
const arr1 = [1, 2, 3];
const arr2 = [...arr1, 4, 5, 6];
6. Promises
Promises are a better way to handle asynchronous operations compared to traditional callbacks. They make your code more readable and manageable, especially when dealing with multiple async processes.
const fetchData = new Promise((resolve, reject) => {
// Simulate async operation
setTimeout(() => resolve('Data fetched!'), 2000);
});
fetchData.then(data => console.log(data));
7. Async/Await
Async/await is built on top of promises and allows you to write asynchronous code that looks synchronous, making it easier to read and debug.
async function getData() {
const data = await fetchData();
console.log(data);
}
getData();
8. Modules (import/export)
ES6 introduced a native module system, allowing you to split your code into reusable modules. You can use `import ` and `export` statements to include or share functionality across different files.
// math.js
export function add(a, b) {
return a + b;
}
// app.js
import { add } from './math.js';
console.log(add(2, 3));
9. Classes
Classes in JavaScript provide a simpler and clearer syntax to create objects and handle inheritance. They’re syntactical sugar over the existing prototype-based inheritance model.
class Animal {
constructor(name) {
this.name = name;
}
speak() {
console.log(`${this.name} makes a sound.`);
}
}
class Dog extends Animal {
speak() {
console.log(`${this.name} barks.`);
}
}
const dog = new Dog('Rex');
dog.speak(); // Rex barks.
10. Let and Const
`**let**` and `**const**` are new ways to declare variables in JavaScript. `let` is block-scoped and prevents variable hoisting issues, while `const` is also block-scoped and is used for variables that shouldn’t be reassigned.
let counter = 1;
const MAX_LIMIT = 100;
if (counter < MAX_LIMIT) {
let message = 'Counter is within the limit';
console.log(message);
}
Conclusion
Understanding and mastering these ES6+ features is crucial for every web developers. They not only make your code more efficient and readable but also help you stay up-to-date with modern JavaScript practices. By incorporating these features into your daily coding routine, you'll improve your productivity and write more maintainable code.
Happy coding!