How to Implement Smooth Scrolling on Your Website with Lenis
Lenis is a lightweight and modern JavaScript library designed to enhance user experience by providing smooth scrolling on websites. Implementing smooth scrolling with Lenis allows developers to create fluid, inertia-based scrolling effects that feel natural and responsive, offering more control over the scrolling behavior compared to standard CSS or JavaScript solutions.
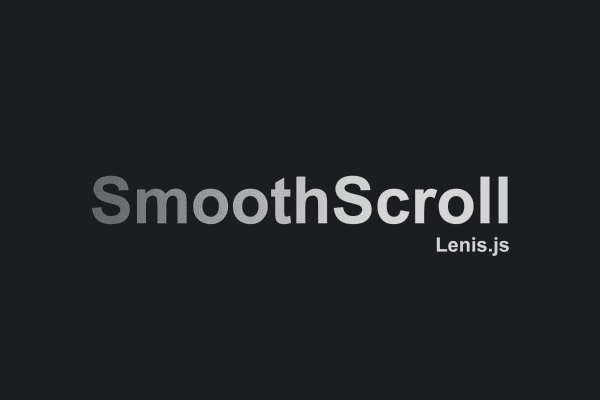
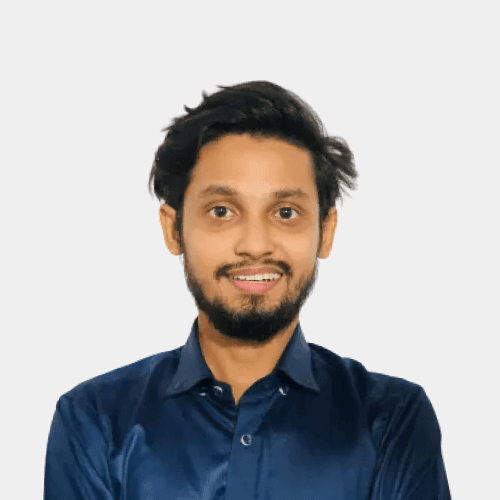
Md Kayesh
6 min readOctober 3, 2024
In this article, we’ll show you how to implement Lenis smooth scrolling on your website, including a step-by-step guide on how to set it up in React and GSAP. By the end, you’ll have a website with smooth, modern scrolling, optimized for a better user experience.
1. Install Lenis
You can add Lenis to your project using npm or by including it through a CDN.
a) Using npm
If you're using a build tool like Webpack or Parcel, you can install Lenis via npm:
npm i lenis
import Lenis from "lenis";
b) Using CDN
If you prefer to use a CDN (Content Delivery Network), you can include the script directly in your HTML file.
<script src="https://unpkg.com/lenis@1.1.13/dist/lenis.min.js"></script>;
2. Initialize Lenis
Once Lenis is installed, you need to initialize it in your JavaScript file.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Lenis Smooth Scroll</title>
</head>
<body>
<!-- Your content here -->
<script src="https://cdn.jsdelivr.net/npm/@studio-freight/lenis"></script>
<script>
// Create a new instance of Lenis
const lenis = new Lenis()
lenis.on('scroll', (e) => {
console.log(e)
})
function raf(time) {
lenis.raf(time)
requestAnimationFrame(raf)
}
requestAnimationFrame(raf)
</script>
</body>
</html>
Recommended CSS:
html.lenis,
html.lenis body {
height: auto;
}
.lenis.lenis-smooth {
scroll-behavior: auto !important;
}
.lenis.lenis-smooth [data-lenis-prevent] {
overscroll-behavior: contain;
}
.lenis.lenis-stopped {
overflow: hidden;
}
.lenis.lenis-smooth iframe {
pointer-events: none;
}
Explanation:
- const lenis = new Lenis() initializes a new Lenis instance.
- The raf function ensures that the smooth scrolling behavior is applied on every frame, creating a smooth animation.
- requestAnimationFrame(raf) starts the smooth scrolling process and continuously updates it.
Using Lenis with GSAP ScrollTrigger:
Using Lenis together with GSAP ScrollTrigger allows you to create highly performant and visually engaging animations while maintaining smooth scrolling. Lenis provides fluid, inertia-based scrolling, while GSAP ScrollTrigger offers fine control over animations tied to scroll events. When combined, they create a polished and modern scrolling experience.
const lenis = new Lenis();
lenis.on("scroll", (e) => {
console.log(e);
});
lenis.on("scroll", ScrollTrigger.update);
gsap.ticker.add((time) => {
lenis.raf(time * 1000);
});
gsap.ticker.lagSmoothing(0);
Using Lenis smooth scroll in your React App
1. lenis/react
lenis/react provides a <ReactLenis>
component that creates a Lenis instance and provides it to its children via context. This allows you to use Lenis in your React app without worrying about passing the instance down through props. It also provides a useLenis
hook that allows you to access the Lenis instance from any component in your app.
Installation
npm i lenis
Basic
import { ReactLenis, useLenis } from "lenis/react";
function Layout() {
const lenis = useLenis(({ scroll }) => {
// called every scroll
});
return <ReactLenis root>{/* content */}</ReactLenis>;
}
GSAP integration in React App
function Component() {
const lenisRef = useRef();
useEffect(() => {
function update(time) {
lenisRef.current?.lenis?.raf(time * 1000);
}
gsap.ticker.add(update);
return () => {
gsap.ticker.remove(update);
};
});
return (
<ReactLenis ref={lenisRef} autoRaf={false}>
{/* content */}
</ReactLenis>
);
}
This is how you can implement smooth scrolling with lenis in your React app. For learn more you can visit here.
Customizing Lenis in React
const lenis = new Lenis({
duration: 1.5, // Scroll duration in seconds
smooth: true, // Enable smooth scrolling
direction: "vertical", // Can be 'vertical' or 'horizontal'
easing: (t) => t * t, // Use quadratic easing
});
By using Lenis for smooth scrolling, you can greatly improve your website’s user experience, especially in React applications. Its lightweight design, inertia-based scrolling, and customizability make it a powerful tool for creating modern, smooth transitions between sections.
Whether you’re building a single-page application (SPA) or a content-heavy website, implementing smooth scrolling with Lenis in React can provide a polished, professional look. With just a few lines of code, your site will be more engaging and easier to navigate.
For learn more about lenis you can visit here