Failed to collect page data for /blogs/[slug] | vercel deployment error solved
In this tutorial we will discuss how to deploy fullStack Next.js App in vercel. We will also fix the error failed to collect data when we use generateStaticProps() in our app.
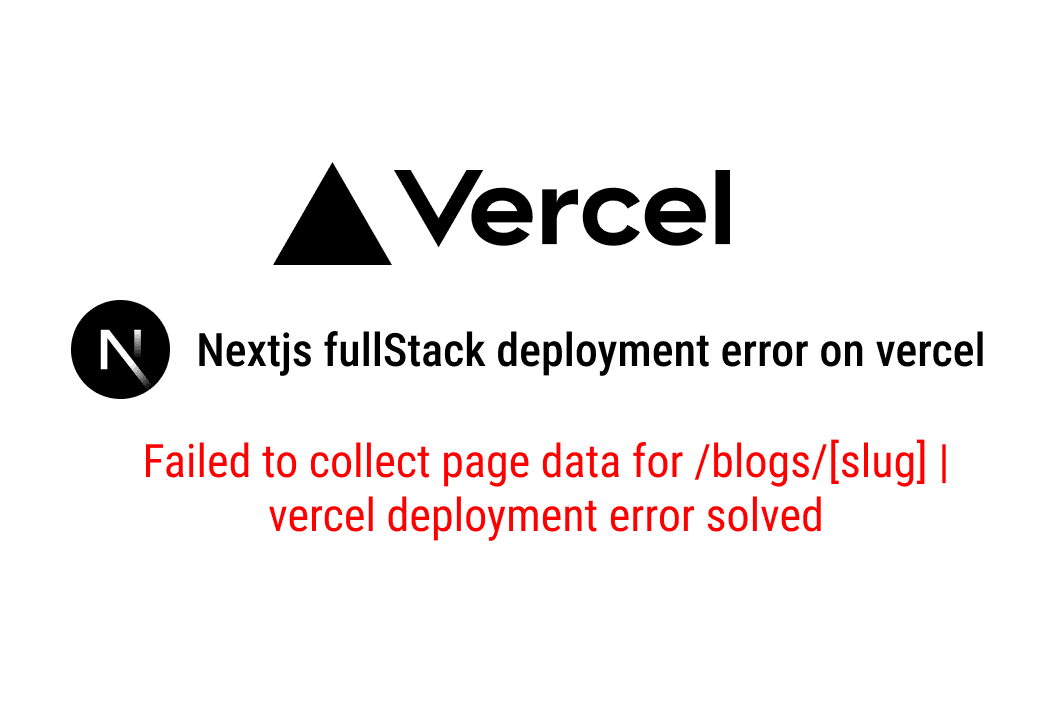
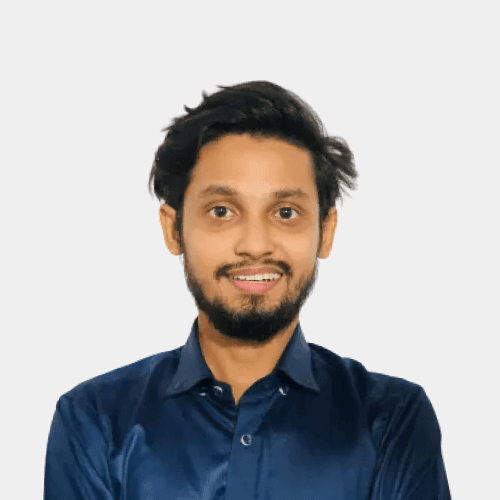
Md Kayesh
6 min readJune 25, 2024
Run yarn build or npm build
Assume that you want to fetch blog posts data with generateStaticParams() for static site generation.
// blogs/[slug]
export async function generateStaticParams() {
const res = await fetchData.get(`https://localhost:3000/api/blogs`);
const blogs = res?.data?.blogs as Blog[];
return blogs.map((post) => ({
slug: post?.slug,
}));
}
const page = async ({ params }: { params: { slug: string } }) => {
const res = await fetchData.get(`/blogs/${params?.slug}`);
const blog = res?.data?.blog as Blog;
return <div>{blog?.title}</div>
You can not run build command when your development server is running on localhost:3000. You have to stop the development server then you run yarn build or npm build in locally you will get this failed to collect page data. Because if your server is not running then generateStaticParams() couldn't fetch the data from the server to get the slugs. This is also happened when we want deploy in the vercel.
Solution
To solve this problem you should comment the generateStaticPrams() then deploy the project on vercel. After deployment finished then uncomment the generateStaticParams() code then again push the updated code on gitHub for deployment. But make sure your API url is as same as deployment url.
// blogs/[slug]
//export async function generateStaticParams() {
// const res = await fetchData.get(`https://localhost:3000/api/blogs`);
// const blogs = res?.data?.blogs as Blog[];
// return blogs.map((post) => ({
// slug: post?.slug,
// }));
//}
const page = async ({ params }: { params: { slug: string } }) => {
const res = await fetchData.get(`/blogs/${params?.slug}`);
const blog = res?.data?.blog as Blog;
return <div>{blog?.title}</div>
This is how you can solve the failed to fetch page data deployment error.